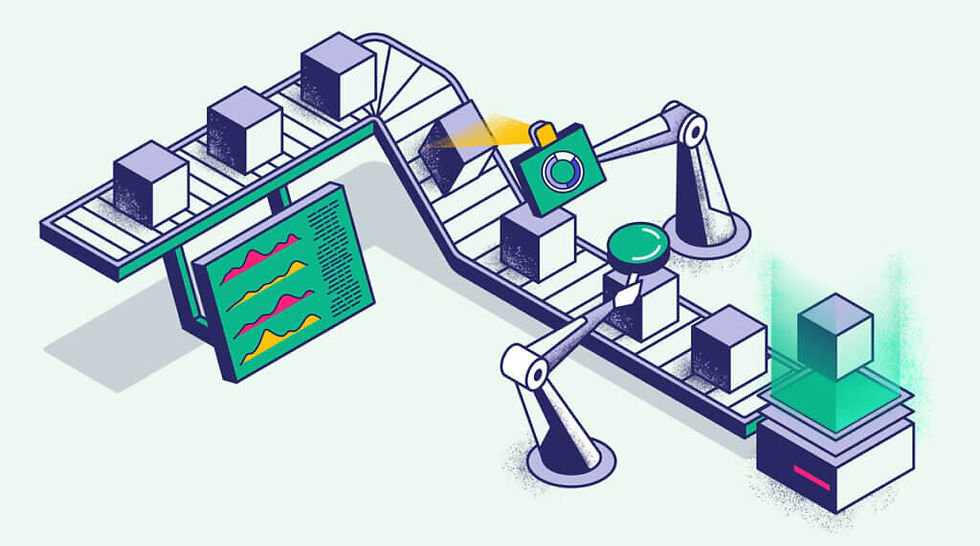
Serverless applications present unique challenges for testing, given their event-driven nature and heavy reliance on managed cloud services. And this is why – after looking at a variety of different serverless approaches in the last few blog posts on modernization – I want to also ensure we have focused discussion on testing serverless applications.
Effective testing approaches for serverless applications must cover various aspects, including unit testing with mocks, integration testing in staging environments, and performance validation through load testing. By implementing a comprehensive testing strategy, teams can mitigate risks, optimize performance, and ensure seamless functionality across diverse cloud environments.
Local Testing and Emulators
Testing serverless functions locally can speed up development cycles and reduce cloud usage costs:
Local Emulators: Use tools like AWS SAM CLI, Azure Functions Core Tools, or Google Cloud Functions Framework to emulate cloud environments on your local machine. These tools simulate the runtime environment, enabling you to test function behavior without deploying to the cloud.
Debugging Locally: Debug serverless functions with tools like Visual Studio Code or JetBrains Rider, which integrate with local emulators to step through code and inspect variables during execution.
Benefits:
Faster feedback loops for developers.
Reduced dependency on live cloud infrastructure during development.
Cost savings by avoiding repeated deployments to test environments.
However, be mindful that local testing may not perfectly replicate cloud behavior, especially for distributed systems or services with managed infrastructure.
Mocking and Integration Testing
Serverless functions often interact with external services, such as databases, APIs, authentication services, and messaging queues. A robust testing strategy must account for both isolated unit tests (mocking dependencies) and end-to-end integration tests (validating real interactions).
Mocking for Unit Tests
Mocking external dependencies ensures that unit tests focus purely on the function’s logic without requiring actual network calls or external service availability.
Approach:
Replace external dependencies (e.g., databases, API clients, or messaging queues) with mock implementations.
Use dependency injection where possible to swap real dependencies with mock objects.
Validate expected interactions, such as function outputs, logging, and error handling.
Tools & Libraries for Mocking:
Java: Mockito for mocking dependencies like AWS SDK clients.
JavaScript/Node.js: Sinon.js for stubbing AWS SDK calls or other API requests.
Python: pytest-mock or Moto to mock AWS services like S3, DynamoDB, and Lambda.
Example (Python with Moto):
If testing an AWS Lambda function that writes data to DynamoDB, we can mock the database interaction – as seen an example below using python:
import boto3
from moto import mock_dynamodb
import pytest
@pytest.fixture
def mock_dynamo():
with mock_dynamodb():
dynamodb = boto3.resource('dynamodb', region_name='us-east-1')
table = dynamodb.create_table(
TableName='TestTable',
KeySchema=[{'AttributeName': 'id', 'KeyType': 'HASH'}],
AttributeDefinitions=[{'AttributeName': 'id', 'AttributeType': 'S'}],
ProvisionedThroughput={'ReadCapacityUnits': 1, 'WriteCapacityUnits': 1}
)
yield table
def test_lambda_function(mock_dynamo):
# Simulate function execution
response = my_lambda_handler({'id': '123', 'data': 'test'}, None)
# Verify expected database interactions
assert response['statusCode'] == 200
This ensures that database interactions are tested without requiring a real AWS DynamoDB table.
Integration Testing in Staging Environments
While unit tests ensure correctness at the function level, integration tests validate how serverless functions interact with real cloud services in a near-production environment.
Approach:
Deploy functions to a staging environment with actual cloud resources.
Perform API tests to verify responses and expected behaviors.
Use event-driven testing (e.g., uploading files to an S3 bucket to trigger Lambda).
Ensure idempotency (re-executing the function with the same input should not cause unintended side effects).
Example (AWS Lambda + S3):
To test a function triggered by an S3 upload:
Deploy Lambda to a staging AWS account.
Upload a test file to an S3 bucket.
Validate the function logs and the downstream effects (e.g., database entry creation).
Tools & Frameworks for Integration Testing:
AWS Step Functions Local: For testing AWS workflows locally before deployment.
Postman/Newman: For automated API testing and request validation.
WireMock: For simulating external APIs in integration tests.
LocalStack: A local AWS environment to test AWS services without cloud deployment.
Balancing Mocking vs. Integration Testing
Mocking is ideal for unit tests, ensuring function logic works without external dependencies.
Integration tests are necessary for verifying interactions with real cloud services and third-party APIs.
By combining both approaches, teams can achieve fast feedback loops during development while ensuring end-to-end reliability before deployment.
Load and Performance Testing
Along with functional testing, load testing is essential for ensuring serverless functions can handle peak traffic efficiently, scale appropriately, and maintain low response times. Since serverless architectures are event-driven and auto-scale dynamically, testing strategies should focus on key performance metrics like cold starts, concurrency limits, and latency.
1. Load Testing Tools
To simulate high traffic and analyse function performance under stress, the following tools can be used:
Artillery – A modern, lightweight load testing tool for APIs and serverless architectures.
k6 – An efficient, developer-friendly load-testing tool that integrates well with CI/CD pipelines.
Apache JMeter – A powerful, widely used tool for performance testing.
Example: Simulating API Gateway Load with Artillery
To test an API Gateway endpoint backed by AWS Lambda, you can use Artillery (code in yaml):
config:
target: "https://your-api-gateway-url.com"
phases:
- duration: 60
arrivalRate: 100 # Simulate 100 requests per second for 60 seconds
scenarios:
- flow:
- get:
url: "/lambda-endpoint"
This simulates thousands of concurrent requests to assess how well the Lambda function scales.
2. Key Metrics to Monitor
Cold Start Times
What it is: The latency introduced when a Lambda function starts a new execution environment after being idle.
How to measure: Use AWS CloudWatch logs or distributed tracing tools like AWS X-Ray.
Optimization: Increase function memory (affects CPU allocation). Keep functions warm using scheduled invocations or provisioned concurrency.
Throughput and Latency
What it is: Measures how many requests the function can process per second and the response time per request.
How to measure: Monitor API Gateway latency, Lambda duration, and overall request response time.
Optimization: Reduce function execution time by optimizing code and dependencies. Use step functions to break large operations into smaller tasks.
Concurrency Limits
What it is: AWS Lambda enforces concurrency limits to prevent overuse of resources.
How to test: Simulate a high number of concurrent executions approaching the AWS service limits.
Optimization: Increase reserved concurrency for critical functions. Use dead-letter queues (DLQs) to handle throttled requests gracefully.
3. Optimizations Based on Load Testing Results
Adjust memory and timeout settings
AWS allocates CPU based on memory settings. Increasing memory can improve execution speed.
Reduce timeout settings for functions that shouldn’t run for long durations.
Refactor function logic
Optimize dependencies (e.g., avoid unnecessary package imports).
Split monolithic Lambda functions into smaller, single-purpose functions.
Use asynchronous processing (e.g., SQS, EventBridge) instead of synchronous API calls.
4. Real-World Load Simulations
Testing should mimic real production traffic patterns, including expected workloads and unexpected spikes.
Example: E-commerce Black Friday Simulation
For an e-commerce platform, simulate a high-traffic sales event by:
Generating requests that mimic real customer behaviour (e.g., browsing, adding to cart, checking out).
Validating auto-scaling behaviour under sudden traffic surges.
Ensuring database and third-party service calls can handle increased demand.
Example: IoT Device Data Processing
For an IoT system where thousands of devices send data every second:
Use k6 to simulate multiple IoT devices sending events to AWS Lambda via IoT Core or Kinesis.
Validate that messages are processed within the expected latency.
Load testing ensures that serverless functions scale efficiently while maintaining low latency and cost-effectiveness. By combining load simulation, monitoring tools, and optimizations, teams can build resilient and high-performing serverless applications.
Conclusion
By combining local testing, dependency mocking, staging integration, and load testing, teams can build resilient serverless applications that scale seamlessly while maintaining high performance and reliability.
Additionally, continuous monitoring and proactive optimizations ensure that serverless functions remain efficient and cost-effective under varying workloads. By leveraging automated testing in CI/CD pipelines, teams can catch performance bottlenecks early and deliver robust, scalable solutions with confidence.
Comments